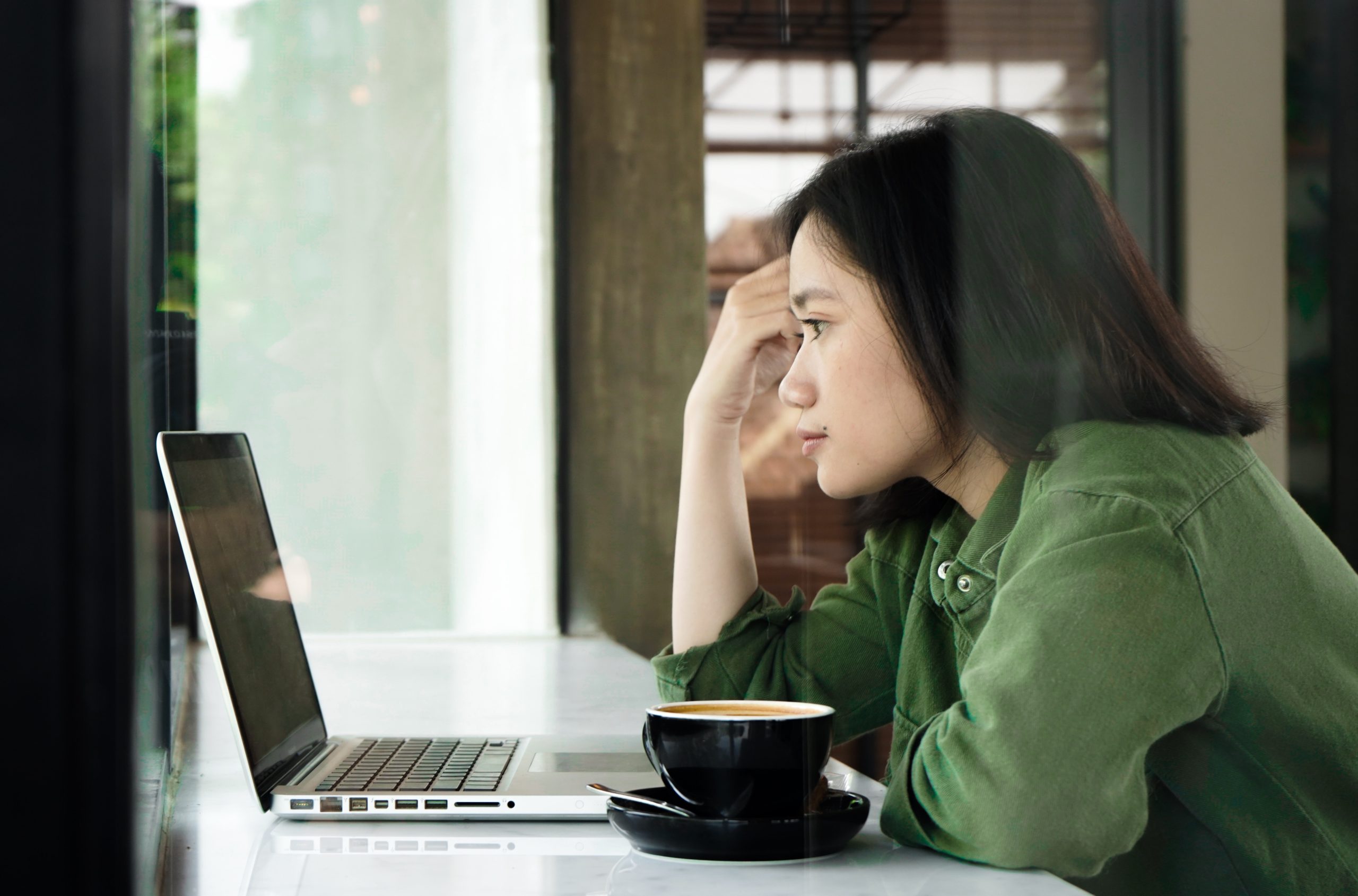
Building a web application from the terminal using the Model-View-Controller (MVC) design pattern can sound daunting at first, but with the right tools and steps, it becomes a straightforward process. This guide provides a comprehensive, step-by-step walkthrough of how to set up an MVC web application purely from the terminal.
What is MVC?
The MVC architecture is a design pattern that separates the application into three components:
- Model: Manages the application’s data and logic.
- View: Handles the representation of data (user interface).
- Controller: Acts as a mediator between the model and the view, processing user input and interacting with the model.
By separating responsibilities, MVC enables developers to write modular, maintainable, and scalable web applications.
Prerequisites
Before following along, ensure that you have the necessary tools installed:
- A terminal or command-line interface (CLI)
- A programming language runtime (such as Node.js, Python, or PHP)
- A basic text editor or IDE (optional but helpful for coding)
This article uses examples in Node.js to demonstrate the process, but the steps can be adapted to other languages and frameworks.
Step-by-Step Guide
1. Initialize the Project
The first step is to create a project directory and initialize it. Open the terminal and enter the following command:
mkdir mvc-app cd mvc-app npm init -y
This will create a new folder for your project (`mvc-app`) and initialize a package.json
file for managing dependencies.
2. Install Dependencies
Next, install the required modules. For a Node.js MVC app, you might need express
for the server and ejs
for templating:
npm install express ejs
You can add additional dependencies like database drivers (e.g., mongoose
for MongoDB or pg
for PostgreSQL) as needed later.
3. Set Up the Directory Structure
Organize your files into meaningful directories from the terminal:
mkdir models views controllers
This command creates three key directories:
- models/: Stores the application’s data and logic.
- views/: Contains templates (e.g.,
.ejs
files) for rendering the UI. - controllers/: Houses the files responsible for managing routes and business logic.
Create the application entry point (e.g., index.js
):
touch index.js
4. Configure the Framework
In index.js
, write the basic configuration for your Express framework:
const express = require("express"); const app = express(); const PORT = 3000; // Set the view engine app.set("view engine", "ejs"); // Define a sample route app.get("/", (req, res) => { res.render("index", { message: "Welcome to the MVC App!" }); }); // Start the server app.listen(PORT, () => { console.log(`Server running on http://localhost:${PORT}`); });
Create a sample views/index.ejs
file to display the content:
<%= message %>
Run the server with:
node index.js
You can now open your browser and navigate to http://localhost:3000
to see the output.
5. Implement Models, Views, and Controllers
Continue building the application by adding specific functionality:
- Model: Write JavaScript or database-specific code to manage data in the
models/
directory. - Controller: Create JavaScript files in
controllers/
to handle business logic and routing. - View: Use
.ejs
,.pug
, or other templating files in theviews/
directory to render data dynamically.
Here is an example of organizing user-related routes in a controller file (controllers/userController.js
):
exports.getUser = (req, res) => { res.render("user", { name: "John Doe" }); };
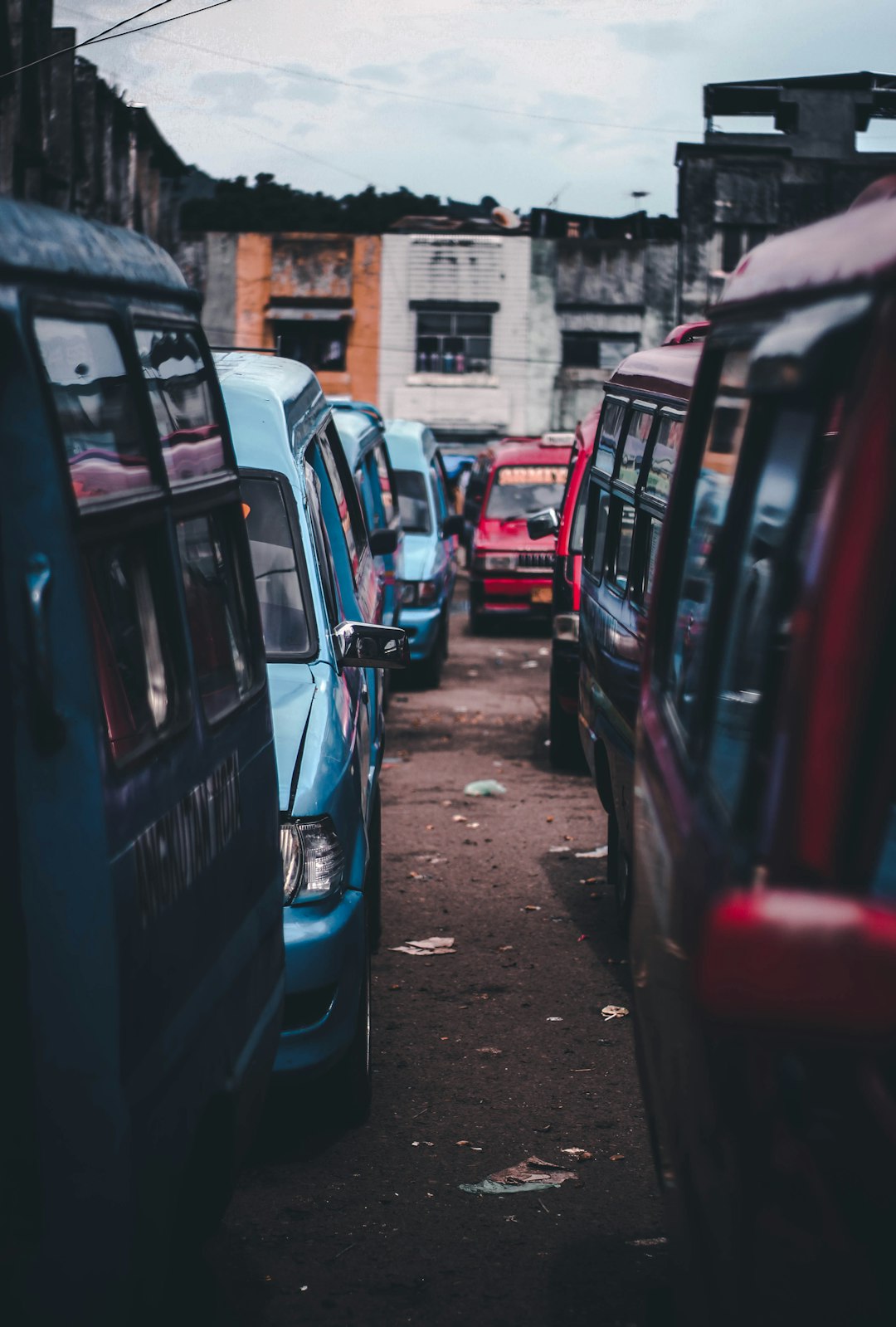
6. Test and Debug
Deploy your app locally and verify that the routes, templates, and data interact as expected. Use debugging tools like console.log()
or browser developer tools for troubleshooting.
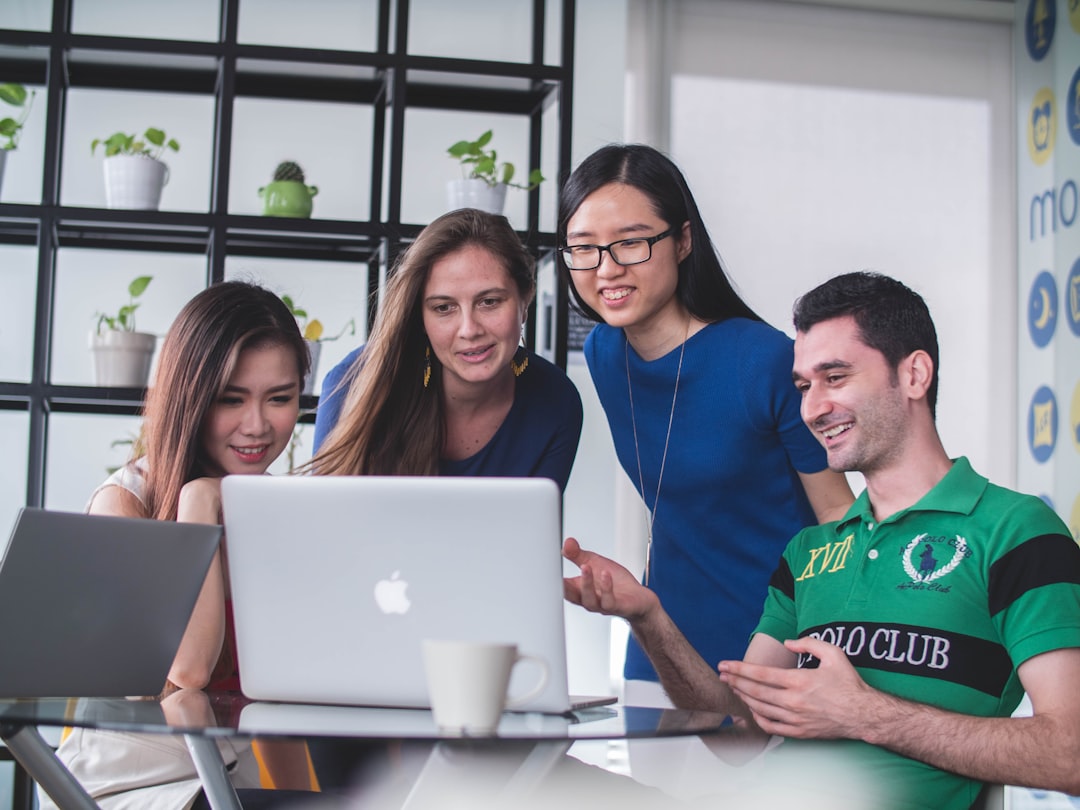
FAQs
- Q: Can this process be adapted to other programming languages?
- A: Absolutely. While this guide uses Node.js as an example, the same principles apply to creating MVC applications in any language, such as Ruby, Python, or PHP.
- Q: Is a terminal-based workflow efficient for large applications?
- A: Yes, though using an IDE with a terminal integration can enhance efficiency by providing additional tools like debugging, linting, and autocomplete.
- Q: How do I deploy this app?
- A: You can deploy the app on platforms like Heroku, AWS, or Vercel. Simply follow the host’s deployment guide and push your files to the target server.
By following this guide, developers can gain confidence in building MVC web apps directly from the terminal, enhancing both their coding skills and understanding of core architectural concepts.