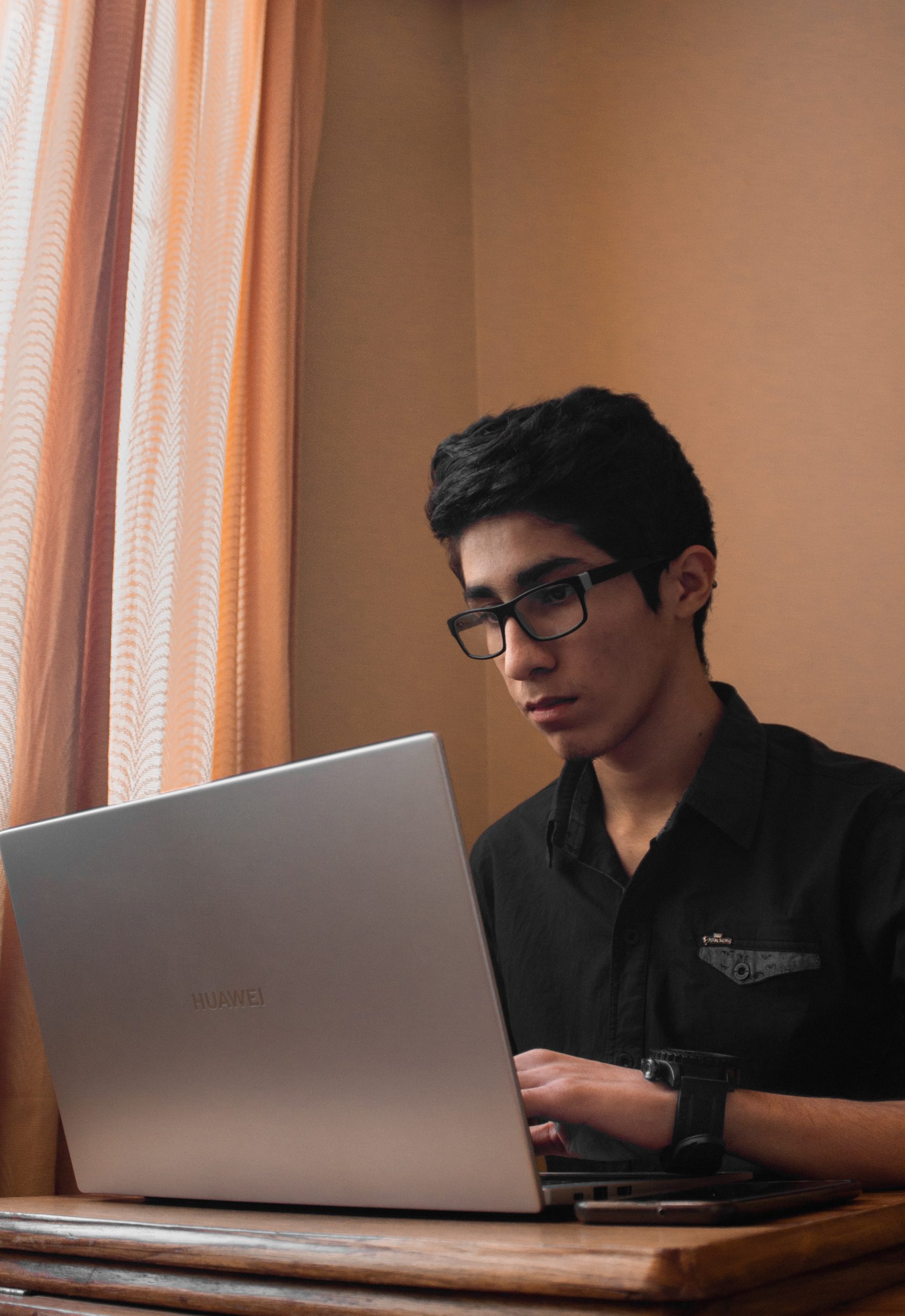
Monitoring file changes and restarting applications automatically is a valuable process, especially for administrators and developers working on file systems or applications requiring constant oversight. PowerShell, a powerful scripting tool for Windows, provides the flexibility to monitor file changes and execute necessary actions, such as restarting an application, whenever updates occur. This article explores how to use PowerShell to monitor file changes and restart applications efficiently.
Understanding the Use Case for Monitoring File Changes
Monitoring file changes is essential in scenarios where critical files or directories are updated frequently, and specific actions must occur in response. For example, in software development, configuration files may change, requiring an application to restart. Similarly, log files, scripts, or data files might need constant monitoring for changes, especially in a production environment.
Using PowerShell, you can automate these tasks by writing scripts that watch for file changes, such as creations, modifications, deletions, or renames, and trigger an action such as restarting an application.
Setting Up File Monitoring with PowerShell
PowerShell provides the FileSystemWatcher class to monitor file system changes. Here is a step-by-step guide to set up file monitoring.
Define the File or Directory to Monitor
To begin, open PowerShell and define the file or folder path you want to monitor. Use the following script as an example:
# Define the folder to monitor
$folderPath = “C:\Path\To\Monitor”
# Create a FileSystemWatcher object
$watcher = New-Object System.IO.FileSystemWatcher
$watcher.Path = $folderPath
$watcher.IncludeSubdirectories = $true
$watcher.EnableRaisingEvents = $true
Here, the FileSystemWatcher object is configured to monitor all changes within the specified directory and its subdirectories.
Specify Events to Monitor
You can configure the watcher to monitor specific events, such as file creations, changes, deletions, or renames. For example:
# Specify event filters
$watcher.NotifyFilter = [System.IO.NotifyFilters]’LastWrite, FileName, DirectoryName’
The NotifyFilter determines which file attributes trigger an event, such as LastWrite for modifications or FileName for renames.
Restarting an Application on File Changes
After setting up the watcher, you can define an action to restart an application whenever a file change occurs. Use the Register-ObjectEvent cmdlet to bind an action to an event:
# Define the application to restart
$appName = “YourAppName.exe”
$appPath = “C:\Path\To\YourAppName.exe”
# Define the action on file change
Register-ObjectEvent $watcher “Changed” -Action {
Write-Host “File change detected. Restarting application…”
Stop-Process -Name $appName -Force -ErrorAction SilentlyContinue
Start-Process -FilePath $appPath
}
Explanation of the Script
Stop-Process: Stops the application process if it is currently running.
Start-Process: Restarts the application using the specified file path.
Register-ObjectEvent: Binds the file change event (Changed) to the defined action.
Running and Testing the Script
Save the script as a PowerShell file (e.g., MonitorFileChanges.ps1) and run it in a PowerShell console with administrator privileges:
.\MonitorFileChanges.ps1
Modify or save a file within the monitored directory to test whether the application restarts successfully when a file change occurs.
PowerShell’s FileSystemWatcher class, combined with event handling, allows you to monitor file changes and take immediate action, such as restarting applications. Automating this process improves efficiency, reduces manual intervention, and ensures your system or application responds dynamically to file updates. Whether for development, administration, or production monitoring, leveraging PowerShell provides a powerful and flexible solution for file change detection and automated task execution.