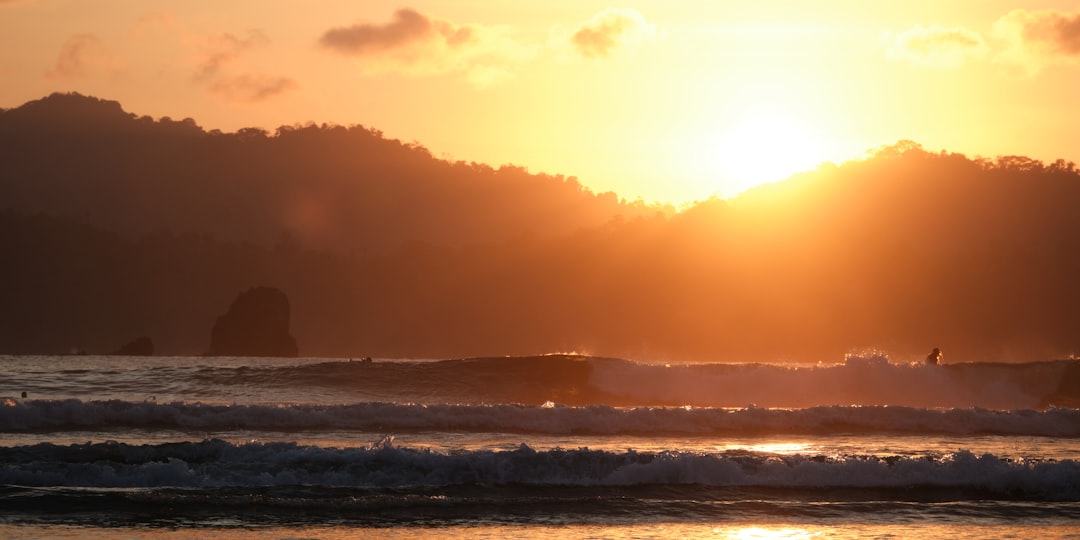
Working with two-dimensional arrays in Java is a common task when handling tabular data or building matrix-based applications. Whether you’re learning Java or developing an enterprise system, you’ll encounter situations where you need to print the contents of a 2D array for debugging, logging, or displaying structured information. In this article, we’ll walk through the most straightforward and efficient ways you can achieve this in Java.
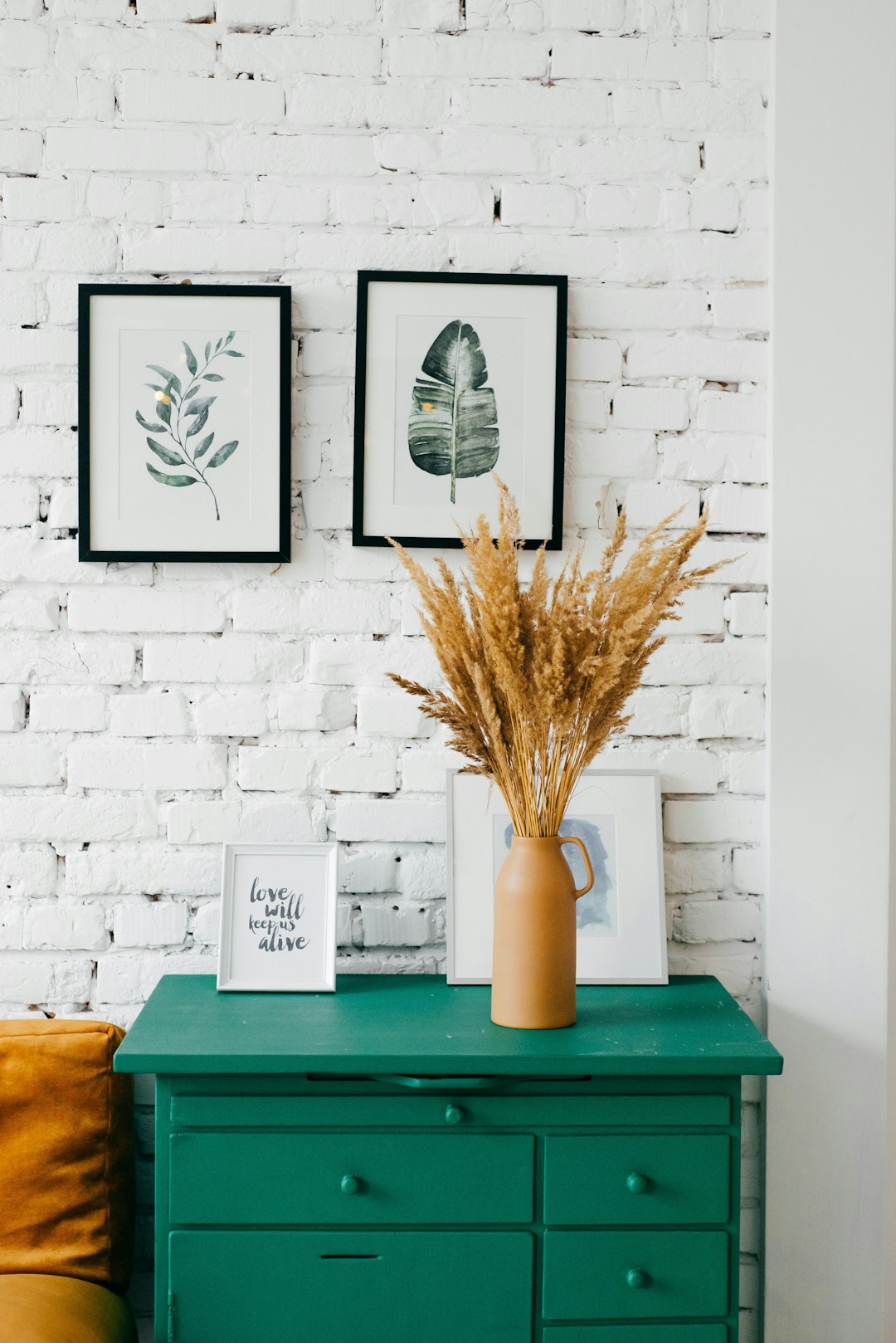
Understanding 2D Arrays in Java
A two-dimensional array is essentially an array of arrays. This structure allows data to be stored in a grid-like format, where each element is accessed using two indices: one for the row and one for the column. Here is how you typically declare and initialize a 2D array in Java:
int[][] matrix = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
Now, to clearly visualize what’s inside this matrix, you need efficient methods to print it out in a readable format.
Method 1: Using Nested For Loops
This is the most traditional and flexible way to print a 2D array. You loop through each row and then each column within that row:
for (int i = 0; i < matrix.length; i++) {
for (int j = 0; j < matrix[i].length; j++) {
System.out.print(matrix[i][j] + " ");
}
System.out.println();
}
Pros:
- Highly customizable: You control every aspect of how data is printed.
- Universally compatible: Works with all types of 2D arrays.
Cons:
- Can be verbose and redundant for simple use cases.
Method 2: Using Enhanced For Loops
Java’s enhanced for loop makes code cleaner and easier to read, especially when you don’t need access to the current index.
for (int[] row : matrix) {
for (int value : row) {
System.out.print(value + " ");
}
System.out.println();
}
This approach is elegant and concise, though it sacrifices a bit of control since you don’t directly access the indices.
Method 3: Using Arrays.deepToString()
If you’re doing quick debugging and don’t care much about formatting, Java provides a built-in way to print multi-dimensional arrays using java.util.Arrays
:
import java.util.Arrays;
System.out.println(Arrays.deepToString(matrix));
This method automatically handles nested structures and displays the data in a structured string format.
Output:
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
Pros:
- Extremely concise – great for debugging.
- No need to write loops.
Cons:
- Not human-friendly for large or user-facing data sets.
- Limited formatting capabilities.
Method 4: Using Helper Methods
For clean and reusable code, you can create a method to handle the printing of any 2D array, whether it’s int[][]
, String[][]
, etc.
public static void print2DArray(int[][] array) {
for (int[] row : array) {
for (int value : row) {
System.out.print(value + "\t");
}
System.out.println();
}
}
Call this method from your main class as follows:
print2DArray(matrix);
[p ai-img]java programming, array function, helper method[/ai-img]
Best Practices When Printing 2D Arrays
Depending on the context, here are a few best practices to make your output clearer and more useful:
- Use tabs or padding to align values, especially for larger datasets.
- Label rows and columns if needed to improve clarity.
- Avoid printing extremely large arrays to the console; truncate or summarize instead.
Conclusion
Printing a 2D array in Java can be as simple or as sophisticated as you need it to be. For quick checks, nested loops or Arrays.deepToString()
are sufficient. For structured output in production-level applications, creating reusable helper methods gives you scalability and better control over formatting. Understanding these methods ensures you’re not only writing efficient Java code but also improving the maintainability and readability of your application’s output.
By mastering these printing techniques, you’re taking a crucial step toward becoming a more effective and professional Java developer.